React Component For Web Speech Api
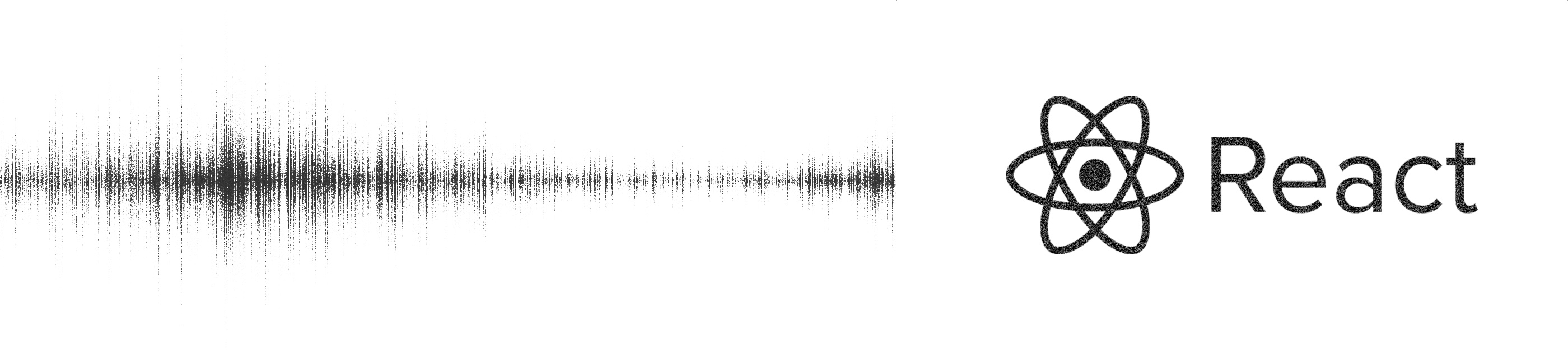
This post demonstrates how to add speech to a react application using the React component for the Web Speech api.
The Web Speech API aims to enable web developers to provide, in a web browser, speech-input
and text-to-speech
output.
The Web Speech API comes in two parts, speech synthesis and speech recognition. This react component supports speech synthesis
, text-to-speech
.
Install
In order to install react-speech
, simply run
$ npm install react-speech --save
Simple Usage
Using react-speech
, is pretty simple, simply React.render
the speech component, setting the text
property, which is rendered to speech.
import React from 'react';
import Speech from 'react-speech';
React.render(
<Speech text="Welcome to react speech" />,
document.getElementById('node')
);
Examples
Here are some examples of using react-speech
Default settings
<Speech
text="I have the default settings" />
Altered my voice
<Speech
text="I have altered my voice"
voice="Google UK English Female" />
Set button colour
const style = {
play: {
button: {
width: '28',
height: '28',
cursor: 'pointer',
pointerEvents: 'none',
outline: 'none',
backgroundColor: 'yellow',
border: 'solid 1px rgba(255,255,255,1)',
borderRadius: 6
},
}
};
<Speech
styles={style}
text="I have changed the colour of the play button and made it smaller" />
Set pitch, rate and volume
<Speech
text="I have altered the pitch, rate and volume of my voice"
pitch="0.5"
rate="0.5"
volume="0.1"
lang="en-GB"
voice="Daniel" />
Set default properties
<Speech
text="I have all properties set to their default"
pitch="1"
rate="1"
volume="1"
lang="en-GB"
voice="Google UK English Male" />
Display pause, stop and resume buttons
<Speech
stop={true}
pause={true}
resume={true}
text="I am displaying all buttons" />
Display text as a button, and override display text
const textstyle = {
play: {
hover: {
backgroundColor: 'black',
color:'white'
},
button: {
padding:'4',
fontFamily: 'Helvetica',
fontSize: '1.0em',
cursor: 'pointer',
pointerEvents: 'none',
outline: 'none',
backgroundColor: 'inherit',
border: 'none'
},
}
<Speech
styles={textstyle}
textAsButton={true}
displayText="Hello"
text="I have text displayed as a button" />
Issues with long text
http://stackoverflow.com/questions/21947730/chrome-speech-synthesis-with-longer-texts
Supported broswers
If a browser does not support Web Speech API
we simply display the text specified. If you are unsure about your browser: